前言
有几周没更新了,用WPF写了一套桌面软件,实现GerBer文件的图像处理,渲染出真实的板卡图像Gerber渲染软件
关于MVVM
MVVM是Model-View-ViewModel的简写,是一种架构模式,其设计目标是从视图层中移除几乎所有的代码,促进UI开发和业务代码的分离,即前后端分离。使得代码的开发、更新和复用更加简单、快捷。
- View 是指可见元素和用户输入功能,包括用户界面、动画和文本。View中的内容不能直接通过交互来更改所呈现的内容,其交互逻辑应当在ViewModel中实现。
- ViewModel位于View层和Model层之间,这里存放了与View交互的代码逻辑,利用Binding命令可将View中的UI元素与ViewModel中的控件连接起来。
- Model是指数据模型,ViewModel能够调用设计好的数据模型。
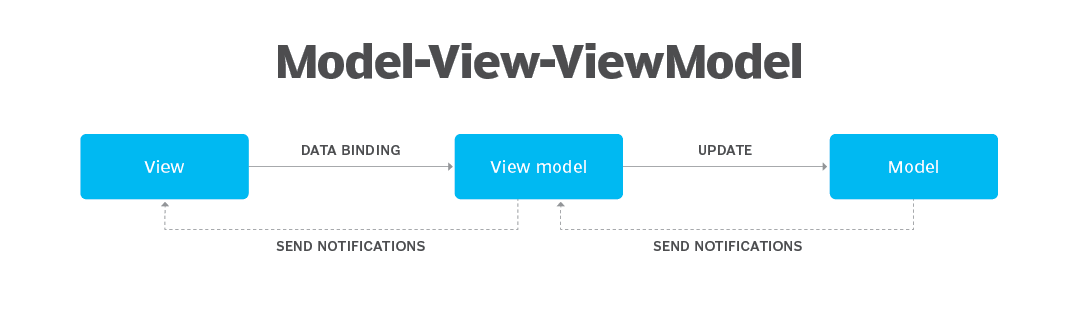
一份简单的MVVM示例
- Model
namespace WpfMvvmExample.Models
{
public class Person
{
public string Name { get; set; } // 存储人名的属性
}
}
- ViewModel
using System.ComponentModel;
using System.Runtime.CompilerServices;
using System.Windows.Input;
using WpfMvvmExample.Models;
namespace WpfMvvmExample.ViewModels
{
public class MainViewModel : INotifyPropertyChanged
{
private Person person = new Person(); // 实例化Person模型
private string greeting; // 用于存储问候语的私有字段
public string Name
{
get { return person.Name; }
set
{
person.Name = value;
OnPropertyChanged(); // 触发属性更改通知
}
}
public string Greeting
{
get { return greeting; }
private set
{
greeting = value;
OnPropertyChanged(); // 触发属性更改通知
}
}
public ICommand ShowGreetingCommand { get; } // 命令,用于显示问候语
public MainViewModel()
{
ShowGreetingCommand = new RelayCommand(ShowGreeting); // 初始化命令并绑定方法
}
private void ShowGreeting()
{
Greeting = $"Hello, {Name}!"; // 设置问候语
}
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged([CallerMemberName] string propertyName = null)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName)); // 通知视图属性已更改
}
}
}
- View
<Window x:Class="WpfMvvmExample.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WpfMvvmExample"
mc:Ignorable="d"
Title="MVVM Example" Height="200" Width="300">
<Window.DataContext>
<local:ViewModels.MainViewModel/> <!-- 设置数据上下文为MainViewModel -->
</Window.DataContext>
<StackPanel Margin="10">
<TextBox Text="{Binding Name, UpdateSourceTrigger=PropertyChanged}" Margin="0,0,0,10"/> <!-- 绑定文本框到Name属性 -->
<Button Content="Say Hello" Command="{Binding ShowGreetingCommand}" Margin="0,0,0,10"/> <!-- 绑定按钮到命令 -->
<TextBlock Text="{Binding Greeting}"/> <!-- 绑定TextBlock到Greeting属性 -->
</StackPanel>
</Window>
- ReadyCommand
在使用MVVM架构开发时,应当实现ICommand接口,这样视图上的元素就可以绑定到命令逻辑上,从而实现前后端的解耦
ReadyCommand 代码:using System;
using System.Windows.Input;
namespace WpfMvvmExample.ViewModels
{
public class RelayCommand : ICommand
{
private readonly Action execute;
private readonly Func<bool> canExecute;
public RelayCommand(Action execute, Func<bool> canExecute = null)
{
this.execute = execute;
this.canExecute = canExecute ?? (() => true); // 如果没有提供canExecute参数,将默认为总是可执行
}
public event EventHandler CanExecuteChanged
{
add { CommandManager.RequerySuggested += value; }
remove { CommandManager.RequerySuggested -= value; }
}
public bool CanExecute(object parameter)
{
return canExecute();
}
public void Execute(object parameter)
{
execute(); // 执行绑定的Action
}
}
}